IOC创建对象的方法
3、IOC创建对象的方法
使用无参构造创建对象,默认!
使用有参构造创建对象
<!--第一种 下标赋值--> <bean id="user" class="com.jan.pojo.User"> <constructor-arg index="0" value="钟健"/> </bean>
类型创建
<!--第二种 通过类型创建 不建议使用--> <bean id="user" class="com.jan.pojo.User"> <constructor-arg type="java.lang.String" value="zhongjian"/> </bean>
参数名
<!-- 第三种 直接通过参数名来设置--> <bean id="user" class="com.jan.pojo.User"> <constructor-arg name="name" value="Jan"/> </bean>
下标赋值
总结:在配置文件加载的时候,容器中的管理的对象就已经初始化了!
4、Spring配置
4.1、别名
<!--别名,如果添加了别名,我们也可以使用别名取到这个对象-->
<alias name="user" alias="userNew"/>
4.2、Bean的配置
<!--
id: bean 的唯一标识符,也就是相当于我们学的对象名
class: bean 对象所对应的全限定名: 包名 + 类型
name: 也是别名, 而且name 可以同时取多个别名
-->
<bean id="userT" class="com.jan.pojo.UserT" name="user2 u2,u3;u4">
<property name="name" value="钟健学习"/>
</bean>
4.3、import
这个import一般用于团队开发使用,他可以将多个配置文件导入合并为一个,假设,现在项目有对人开发,将三人不同的开类需注册到不同的bean中,我们可以利用import将所有人的beans.xml合并为一个总的!
张三
李四
王五
applicationContext
<import resource="beans.xml"/> <import resource="beans2.xml"/> <import resource="beans3.xml"/>
5、依赖注入
5.1、构造器注入
依赖注入:
依赖:bean对象的创建依赖于容器!
注入: bean对象中的所有属性,由容器注入!
【环境搭建】
复杂类型
public class Address { private String address; public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } }
真实测试对象
public class Student { private String name; private Address address; private String[] books; private List<String>hobbys; private Map<String,String> card; private Set<String> games; private String wife; private Properties info; }
beans.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="student" class="com.jan.pojo.Student"> <!--第一种,普通注入,value--> <property name="name" value="钟健"/> </bean> </beans>
测试类
public class MyTest { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml"); Student student = (Student) context.getBean("student"); System.out.println(student.getName()); } }
完善注入信息
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="address" class="com.jan.pojo.Address"> <property name="address" value="北京"/> </bean> <bean id="student" class="com.jan.pojo.Student"> <!--第一种,普通注入,value--> <property name="name" value="钟健"/> <!--第二种,bean注入,ref--> <property name="address" ref="address"/> <!--数组注入--> <property name="books"> <array> <value>红楼梦</value> <value>水浒传</value> <value>三国演义</value> <value>西游记</value> </array> </property> <!--List--> <property name="hobbys"> <list> <value>听歌</value> <value>敲代码</value> <value>看电影</value> </list> </property> <!--Map--> <property name="card"> <map> <entry key="身份证" value="111111222222223333"/> <entry key="银行卡" value="123456789"/> </map> </property> <!--Set--> <property name="games"> <set> <value>LOL</value> <value>COS</value> </set> </property> <!--null--> <property name="wife"> <null/> </property> <!--Properties--> <property name="info"> <props> <prop key="driver">20230102</prop> <prop key="url">男</prop> <prop key="username">root</prop> <prop key="password">123456</prop> </props> </property> </bean></beans>
5.2、Set方式注入【重点】
5.3、扩展方式注入
我们可以使用P命名空间和C 命名空间进行注入
官方解释
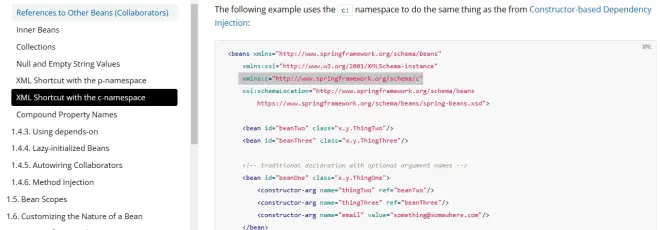
使用
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:c="http://www.springframework.org/schema/c" xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- P命名空间注入,可以直接注入属性的值 property--> <bean id="user" class="com.jan.pojo.User" p:name="钟健" p:age="18"/> <!-- c命名空间注入,通过构造器注入 construct-args--> <!--在User类中要有无参和有参构造器才可以使用 C 命名注入--> <bean id="user2" class="com.jan.pojo.User" c:age="18" c:name="Jan"/> </beans>
测试
public void test2(){ ApplicationContext context = new ClassPathXmlApplicationContext("userbeans.xml"); //User user = context.getBean("user", User.class); User user = context.getBean("user2", User.class); System.out.println(user); }
注意点: P 命名和 C 命名空间不能直接使用,需要导入xml约束!
P命名注入
xmlns:p="http://www.springframework.org/schema/p"
C命名注入
xmlns:c="http://www.springframework.org/schema/c"
5.4、bean的作用域
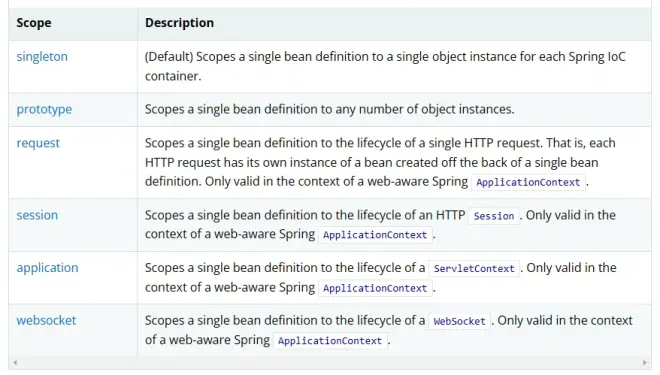
单例模式(Spring默认机制)--->30分钟的教学记得看
<bean id="user2" class="com.jan.pojo.User" c:age="18" c:name="Jan" scope="singleton"/>
有时候并发情况下会产生延迟或数据不一致,单线程一般用这个
原型模式:每次从容器中get的时候,都会产生一个新对象!
<bean id="user2" class="com.jan.pojo.User" c:age="18" c:name="Jan" scope="prototype"/>
别特浪费资源,多线程可以使用原型模式
其余的request、session、application,这些个只能在web开发中使用的!
6、Bean的自动配置
自动配置是Spring满足bean依赖的一种方式
Spring会在上下文中自动寻找,并自动给bean装配属性!
在spring的三种配置方式:
在xml中显示的配置
在java中显示的配置
隐式 的自动装配bean【重要】
6.1、测试
环境搭建:一个人有两个宠物!
原来的xml配置
<bean id="cat" class="com.jan.pojo.Cat"/>
<bean id="dog" class="com.jan.pojo.Dog"/>
<bean id="people" class="com.jan.pojo.People">
<property name="name" value="钟健"/>
<property name="cat" ref="cat"/>
<property name="dog" ref="dog"/>
</bean>
6.2、ByName自动装配
<bean id="cat" class="com.jan.pojo.Cat"/><bean id="dog" class="com.jan.pojo.Dog"/><!--
ByName: 会自动在容器上下文中查找,和自己对象set方法后面的值对应的 bean id!
ByType: 会自动在容器上下文中查找,和自己对象属性类型相同的 bean !
--><bean id="people" class="com.jan.pojo.People" autowire="byName">
<property name="name" value="钟健"/></bean>
6.3、ByType自动装配
<bean class="com.jan.pojo.Cat"/><bean class="com.jan.pojo.Dog"/><!--
ByName: 会自动在容器上下文中查找,和自己对象set方法后面的值对应的 bean id!
ByType: 会自动在容器上下文中查找,和自己对象属性类型相同的 bean !
--><bean id="people" class="com.jan.pojo.People" autowire="byType">
<property name="name" value="钟健"/></bean>
小结:
byname的时候,需要保证所有的bean的id唯一,并且这个bean需要和自动注入的属性的set方法的值一致!
bytype的时候,需要保证所有的bean的class唯一,并且这个bean需要和自动注入的属性的类型一致!