C Primer Plus Test 5
试题来源:C Primer Plus (第6版)中文版
本章主要为运算符、表达式和语句的测试题。
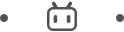
5.1 编写一个程序,把分钟表示的时间转换成用小时和分钟表示的时间。使用#define或const创建一个表示60的符号常量或const变量。通过while循环让用户重复输入值,直到用户输入小于或等于0的值才停止循环。
#include<stdio.h>
#include<stdlib.h>
#define TIME 60
int main(){
int min, h, m;
printf("Enter the minute:\n");
scanf("%d", &min);
while(min > 0){
h = min / TIME;
m = min % TIME;
printf("The time is %d hour %d minute\n", h, m);
printf("Enter the minute:\n");
printf("If you want to stop it, enter 0 or less 0\n");
scanf("%d", &min);
}
system("pause");
return 0;
}
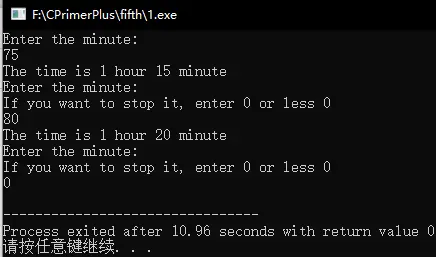
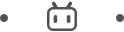
5.2编写一个程序,提示用户输入一个整数,然后打印从该数到比该数大10的所有整数(例如,用户输入5,则打印5~15的所有整数,包括5和15)。要求打印的各值之间用一个空格、制表符或换行符分开。
#include<stdio.h>
#include<stdlib.h>
int main(){
int num, max;
printf("Enter one number:\n");
scanf("%d",&num);
max = num + 10;
printf("空格:");
while( num <= max){
printf("%d ",num);
num ++;
}
printf("\n");
num = max - 10;
printf("制表符:");
while( num <= max){
printf("%d\t",num);
num ++;
}
printf("\n");
num = max - 10;
printf("换行符:");
while( num <= max){
printf("%d\n",num);
num ++;
}
system("pause");
return 0;
}
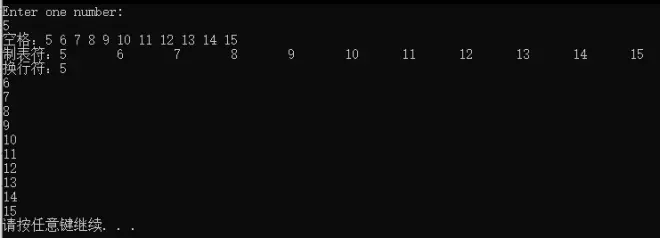
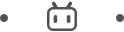
5.3编写一个程序,提示用户输入天数,然后将其转换成周数和天数。例如用户输入18,则转换成2周4天。以下面的格式显示结果:
18 days are 2 weeks, 4 days.
通过while循环让用户重复输入天数,当用户输入一个非正值时(如0或-20),循环结束。
#include<stdio.h>
#include<stdlib.h>
#define W_D 7
int main(){
int day, weeks, days;
printf("Enter the number of day:\n");
scanf("%d", &day);
while(day > 0){
weeks = day / W_D;
days = day % W_D;
printf("%d days are %d weeks, %d days.\n", day, weeks, days);
printf("Enter the number of day:(<=0 to quit)\n");
scanf("%d", &day);
}
system("pause");
return 0;
}
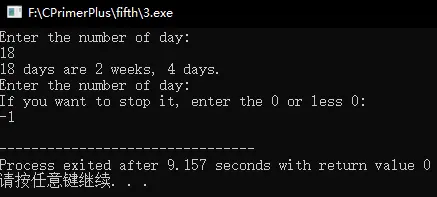
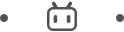
5.4编写一个程序,提示用户输入一个身高(单位:厘米),并分别以厘米和英寸为单位显示该值,允许有小数部分。程序应该能让用户重复输入身高,直到用户输入一个非正值。其输出示例如下:
Enter a height in centimeters: 182
182.0 cm = 5 feet, 11.7 inches
Enter a height in centimeters (<=0 to quit): 168.7
168.7 cm = 5 feet, 6.4 inches
Enter a height in centimeters (<=0 to quit): 0
bye
#include<stdio.h>
#include<stdlib.h>
#define C_F 0.0328
#define F_I 12
int main(){
float height, inches, c_f, feet;
int feets;
printf("Enter a height in centimeters:");
scanf("%f", &height);
while(height > 0){
feet = height * C_F;
feets = (int)feet;
inches = (feet - feets) * F_I;
printf("%.1f cm = %d feet, %.1f inchces\n", height, feets, inches);
printf("Enter a height in centimeters(<=0 to quit):\n");
scanf("%f", &height);
}
printf("bye\n");
system("pause");
return 0;
}
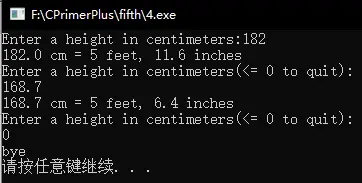
5.5修改程序addemup.c,你可以认为addmup.c是计算20天里赚多少钱的程序(假设第1天赚$1、第2天赚$2,以此类推)。修改程序,使其可以与用户交互,根据用户输入的数进行计算(即,用读入的一个变量来代替20)。
addemup.c
#include<stdio.h>
int main(){
int count, sum;
count = 0;
sum = 0;
whlle(count ++ < 20)
sum = sum + count;
printf("sum=%d\n",sum);
return 0;
}
#include<stdio.h>
#include<stdlib.h>
int main(){
int sum, num, count;
sum = 0;
printf("Enter a number:\n");
scanf("%d", &num);
count = 0;
while(count <= num){
sum = sum + count;
count = count + 1;
}
printf("The end is %d\n", sum);
system("pause");
return 0;
}
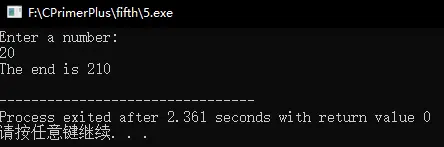
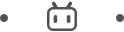
5.6修改编程练习5.5的程序,使其能计算整数的平方和(可以认为第1天赚$1、第2天赚$4),以此类推。C没有平方函数,但是可以用n*n来表示n的平方。
#include<stdio.h>
#include<stdlib.h>
int main(){
int sum, count;
sum = 0;
printf("Enter a number:\n");
scanf("%d", &num);
count = 0;
while(count ++ < num){
sum += count * count;
}
printf("%d\n", sum);
system("pause");
return 0;
}
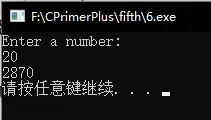
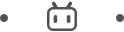
5.7编写一个程序,提示用户输入一个double类型的数,并打印该数的立方值。自己设计一个函数计算并打印立方值。main()函数要把用户输入的值传递给该函数。
#include<stdio.h>
#include<stdlib.h>
double Cbue(double num){
return (num * num * num);
}
int main(){
double num;
printf("Enter a num of double:\n");
scanf("%lf", &num);
printf("cbue is %lf\n", Cbue(num));
return 0;
}
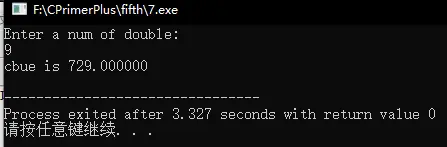
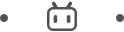
5.8编写一个方程,显示求模运算的结果。把用户输入的第一个整数作为
求模运算符的第二个运算对象,该数在运算过程中保持不变。用户输入
的书是第一个运算对象。当用户输入一个非正值时,程序结束。
输出示例如下:
This program computes moduli.
Enter an integer to serve as the second operand:256
Now enter the first operand:438
438 % 256 is 182
Enter next number for first operand (<=0 to quit):1234567
1234567 % 256 is 135
Enter next number for first operand (<=0 to quit):0
Done
#include<stdio.h>
#include<stdlib.h>
int main(){
int num,f_oper,mod;
printf("This program computes moduli.\n");
printf("Enter an integer to serve as the second operand:");
scanf("%d",&num);
printf("\nNow enter the first operand:");
scanf("%d",&f_oper);
while(f_oper > 0){
mod = f_oper % num;
printf("\n%d %% %d is %d",f_oper,num,mod);
printf("\nEnter next number for first operand (<=0 to quit):");
scanf("%d",&f_oper);
}
printf("Done\n");
return 0;
}
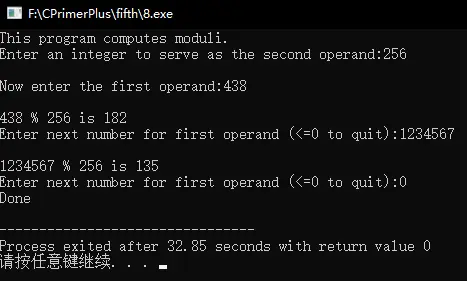
5.9编写一个程序,要求用户输入一个华氏温度。程序应读取double
类型的值作为温度值,并把该值作为参数传递给一个用户自定义的
函数Temperatures()。该函数计算摄氏温度和开氏温度,并以小数
点后面两位数的精度显示3种温度。要使用不同的温标来表示这3个
温度。下面是华氏温度转摄氏温度的公式:
摄氏温度 = 5.0/9.0*(华氏温度 - 32.0)
开氏温度常用于科学研究,0表示绝对零,代表最低的温度。下面
是摄氏温度转开氏温度的公式:
开氏温度 = 摄氏温度 + 273.16
Temperatures()函数中用const创建温度转换中使用的变量。在main
函数中使用一个循环让用户重复输入温度,当用户输入q或其他非数字
时,循环结束。scanf函数返回读取数据的数量,所以如果读取数字则
返回1,如果读取q则不返回1.可以使用==运算符将scanf的返回值和1
作比较,测试两值是否相等。
#include<stdio.h>
#include<stdlib.h>
double Temperatures(double tem){
const double h_s = 5.0/9.0;
const double s_k = 273.16;
double s_tem, k_tem;
s_tem = (tem - 32.0) * h_s;
k_tem = s_tem + s_k;
printf("%.2lf du\t %.2lf kai\n",s_tem,k_tem);
}
int main(){
double tem;
int status;
printf("Enter a number of huashi:");
status = scanf("%lf",&tem);
while(status == 1){
printf("\n");
Temperatures(tem);
printf("Enter a number of huashi('q' to quit):");
status = scanf("%lf",&tem);
}
printf("Bye\n");
system("pause");
return 0;
}
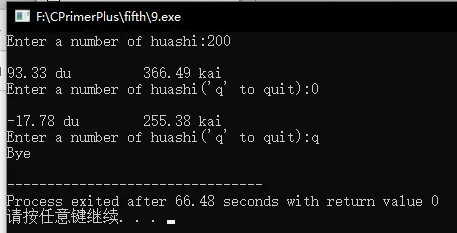