JSP例子等:三元运算符,JSP四大作用域,EL表达式,$,页面跳转,转发【诗书画唱】
1、创建一个Student对象,里面包含有姓名sname(String),爱好hobby(String),生日birth(Date),性别ssex(char),班级scls(String)五个属性,在stu.jsp页面通过EL表达式将学生的详细信息显示页面上

package com.SSHC;
import java.util.Date;
public class Student {
private String sname;
private String hobby;
private Date birth;
private char ssex;
private String scls;
public Student(){
}
public String getSname() {
return sname;
}
public void setSname(String sname) {
this.sname = sname;
}
public String getHobby() {
return hobby;
}
public void setHobby(String hobby) {
this.hobby = hobby;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
public char getSsex() {
return ssex;
}
public void setSsex(char ssex) {
this.ssex = ssex;
}
public String getScls() {
return scls;
}
public void setScls(String scls) {
this.scls = scls;
}
public Student(String sname, String hobby, Date birth, char ssex, String scls) {
super();
this.sname = sname;
this.hobby = hobby;
this.birth = birth;
this.ssex = ssex;
this.scls = scls;
}
@Override
public String toString() {
return "Student [sname=" + sname + ", hobby=" + hobby + ", birth=" + birth
+ ", ssex=" + ssex + ", scls=" + scls + "]";
}
}
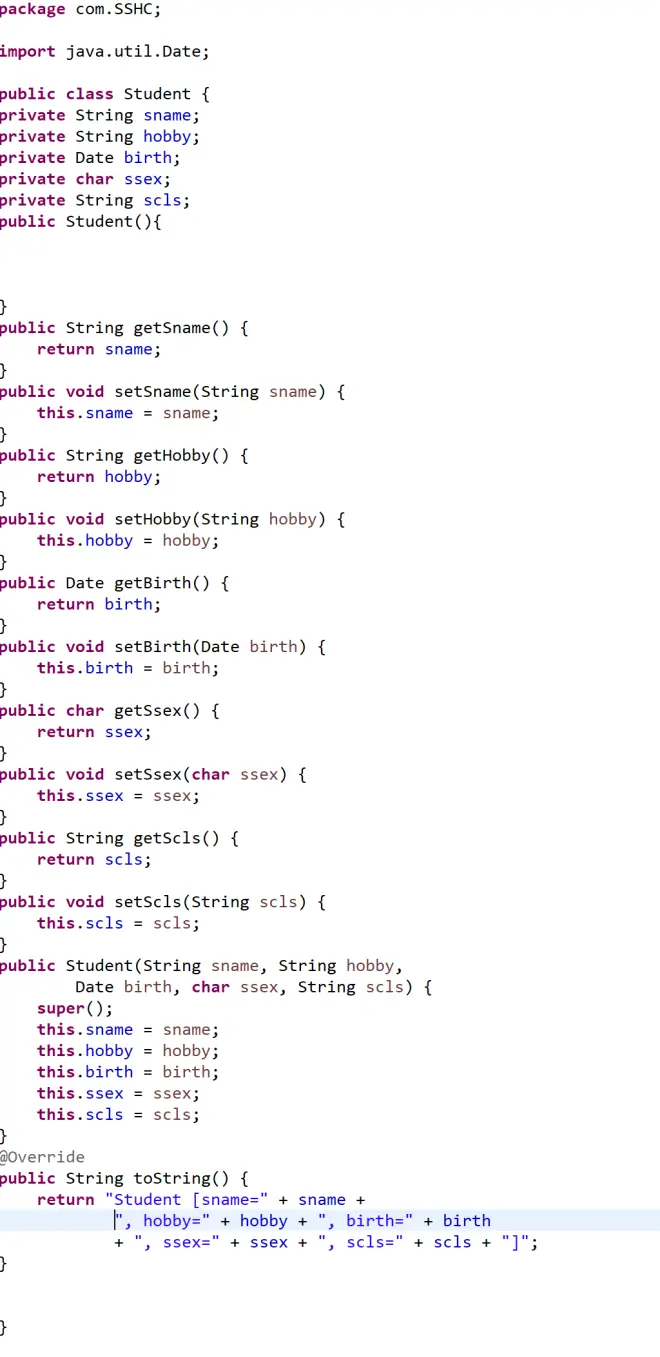

<%@page import="com.SSHC.Student"%>
<%@page import="java.util.Date"%>
<%@ page language="java" contentType="text/html;
charset=UTF-8" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"
+request.getServerName()+":"+request.getServerPort()+path+"/";
Student S = new Student();
S.setSname("诗书画唱");
S.setHobby("做视频和编程");
//下面的Date的类型要声明为“X/X/X”的格式,不然会报错等。
Date D= new Date("2000/7/20");
S.setBirth(D);
S.setSsex('男');
S.setScls("诗书画唱班");
request.setAttribute("KeyS", S);
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base hreff="<%=basePath%>">
<title></title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content=
"keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<h1>用“.”调用的方法调用属性,姓名:${KeyS.sname }</h1>
<h1>用“[]”调用的方法调用属性,爱好:${KeyS["hobby"] }</h1>
<h1>用“.”调用的方法调用属性,生日:${KeyS.birth }</h1>
<h1>用“[]”调用的方法调用属性,性别:${KeyS["ssex"] }</h1>
<h1>用“.”调用的方法调用属性,班级:${KeyS.scls }</h1>
</body>
</html>
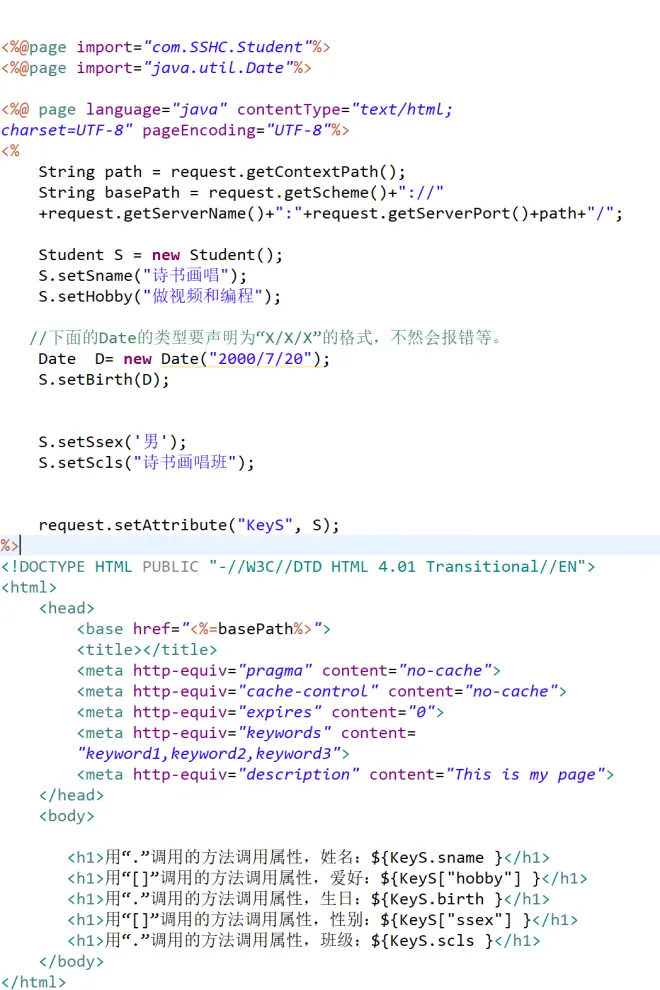
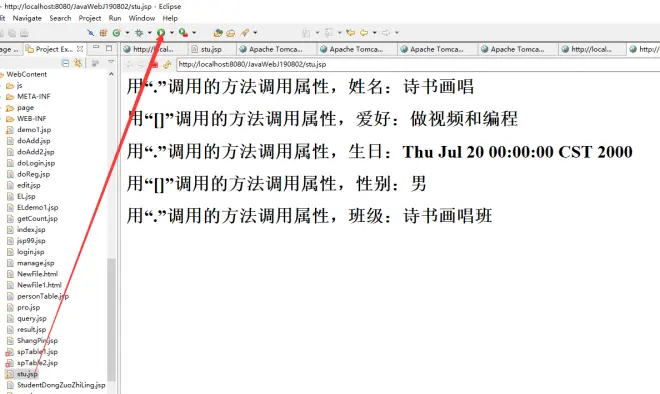
2、创建Car汽车类,包含品牌,价格属性,通过a.jsp页面跳转到b.jsp页面,在a.jsp页面创建Car对象,在b.jsp页面通过EL表达式打印出汽车的品牌和价格。
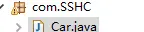
package com.SSHC;
public class Car {
private String pinPai;
private Double price;
public String getPinPai() {
return pinPai;
}
public void setPinPai(String pinPai) {
this.pinPai = pinPai;
}
public Double getPrice() {
return price;
}
public void setPrice(Double price) {
this.price = price;
}
}
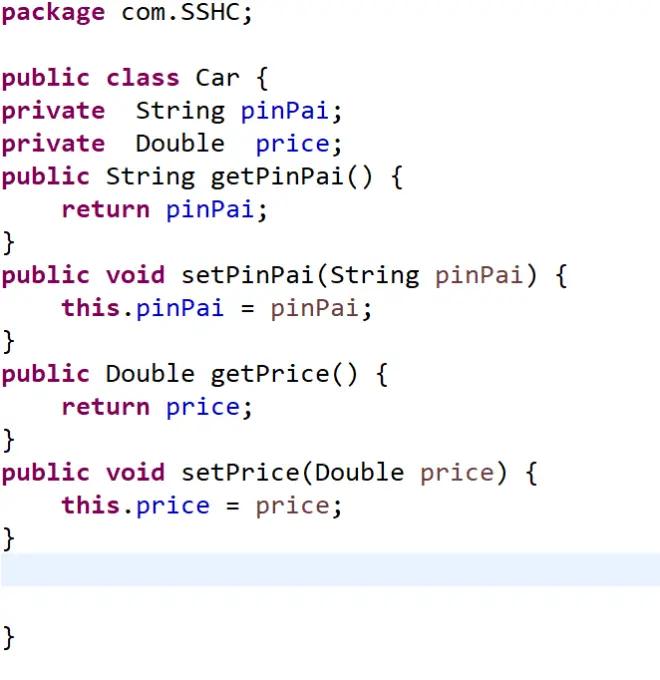
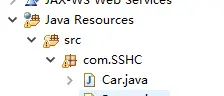
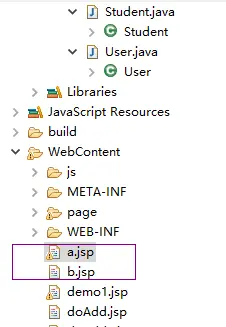

<%@page import="com.SSHC.Student"%>
<%@page import="java.util.Date"%>
<%@ page language="java" contentType="text/html;
charset=UTF-8" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"
+request.getServerName()+":"+request.getServerPort()+path+"/";
Student S = new Student();
S.setSname("诗书画唱");
S.setHobby("做视频和编程");
//下面的Date的类型要声明为“X/X/X”的格式,不然会报错等。
Date D= new Date("2000/7/20");
S.setBirth(D);
S.setSsex('男');
S.setScls("诗书画唱班");
request.setAttribute("KeyS", S);
request.getRequestDispatcher("b.jsp")
.forward(request, response);
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base hreff="<%=basePath%>">
<title></title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content=
"keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
</body>
</html>
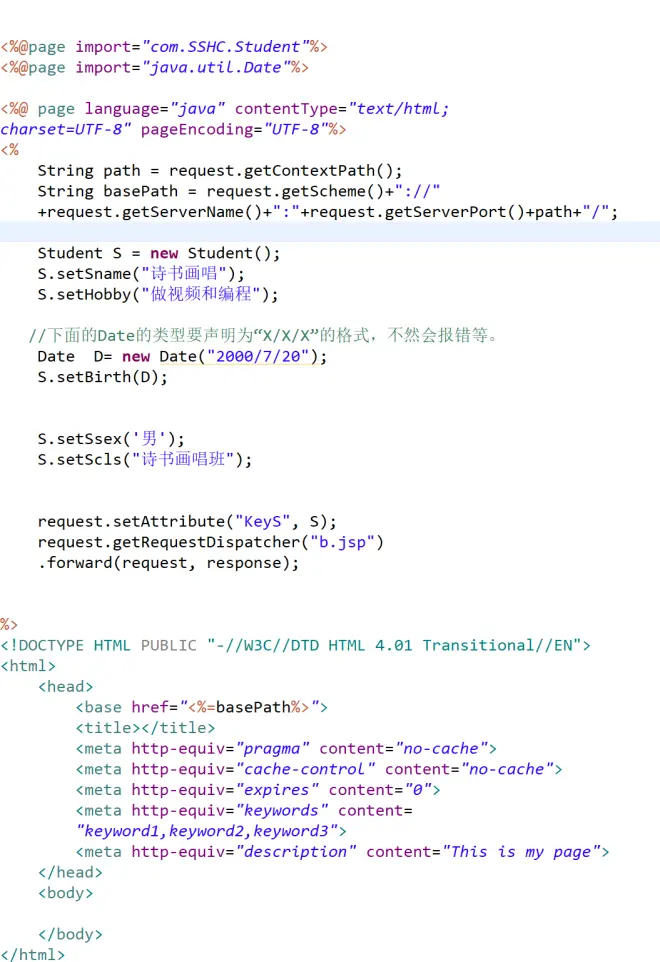

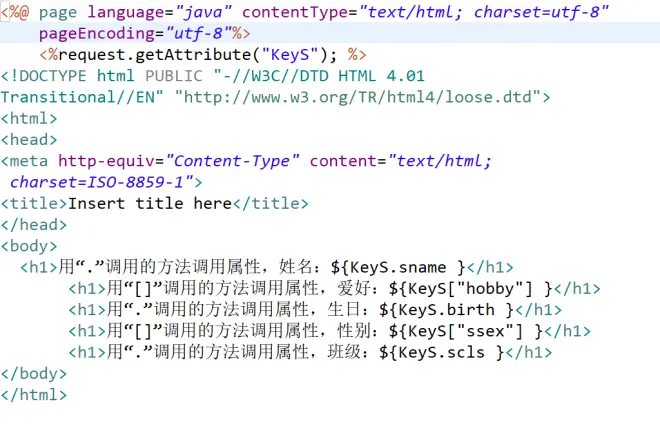
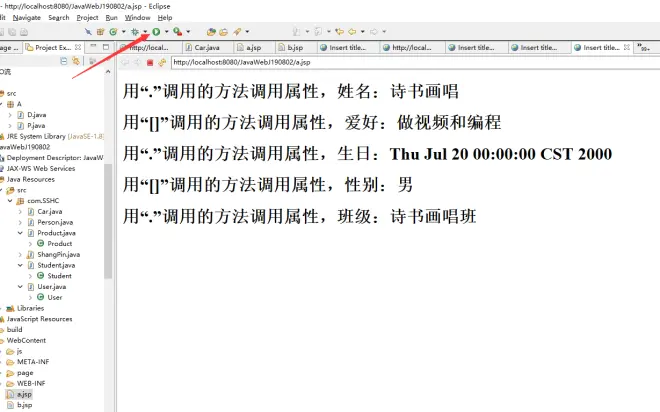
3、创建一个Product商品类,包含商品名称、商品价格,商品类型,通过my.jsp跳转到you.jsp页面,在my.jsp页面创建一个商品类,在you.jsp页面通过EL表达式显示出商品的所有信息。
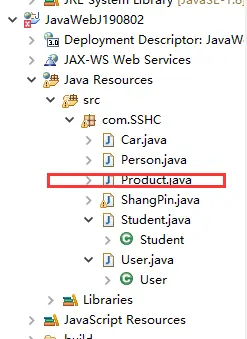
package com.SSHC;
public class Product {
private String name;
private Double price;
private String type;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Double getPrice() {
return price;
}
public void setPrice(Double price) {
this.price = price;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
}
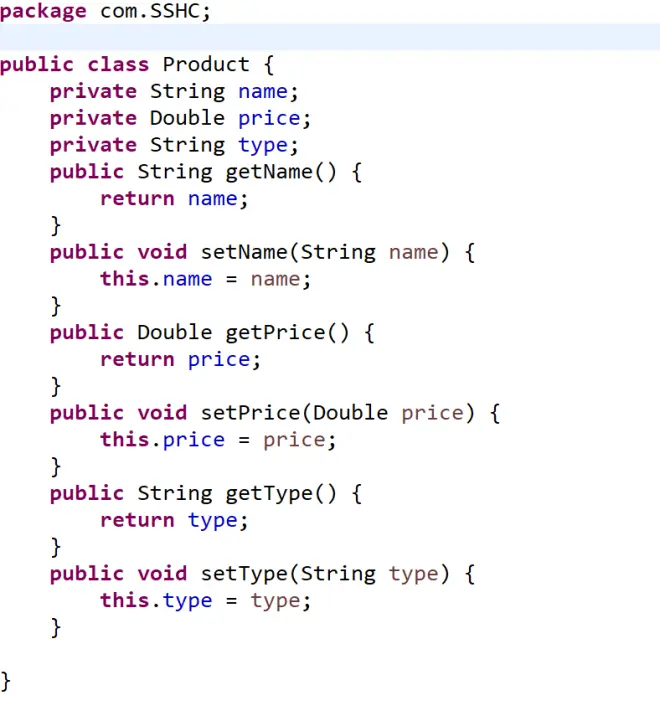

<%@page import="com.SSHC.Product"%>
<%@page import="java.util.Date"%>
<%@ page language="java" contentType="text/html;
charset=UTF-8" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"
+request.getServerName()+":"+request.getServerPort()+path+"/";
Product S = new Product();
S.setName("诗书画唱");
S.setPrice(2.33);
S.setType("CD类");
request.setAttribute("KeyS", S);
request.getRequestDispatcher("you.jsp")
.forward(request, response);
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base hreff="<%=basePath%>">
<title></title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content=
"keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
</body>
</html>
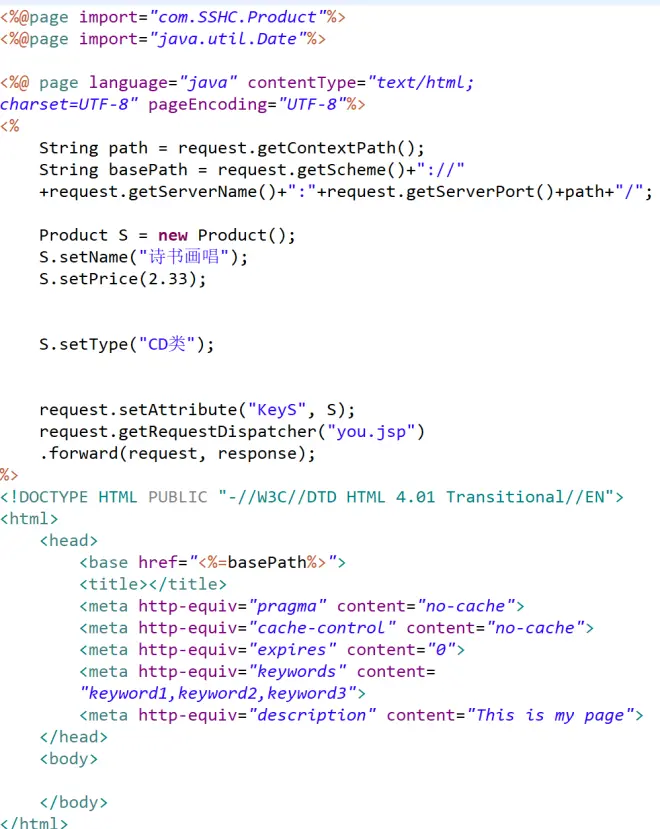

<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<%request.getAttribute("KeyS"); %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01
Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;
charset=ISO-8859-1">
<title>Insert title here</title>
</head>
<body>
<h1>用“.”调用的方法调用属性,商品名称:${KeyS.name }</h1>
<h1>用“[]”调用的方法调用属性,商品价格:${KeyS["price"] }</h1>
<h1>用“.”调用的方法调用属性,商品类型:${KeyS.type }</h1>
</body>
</html>
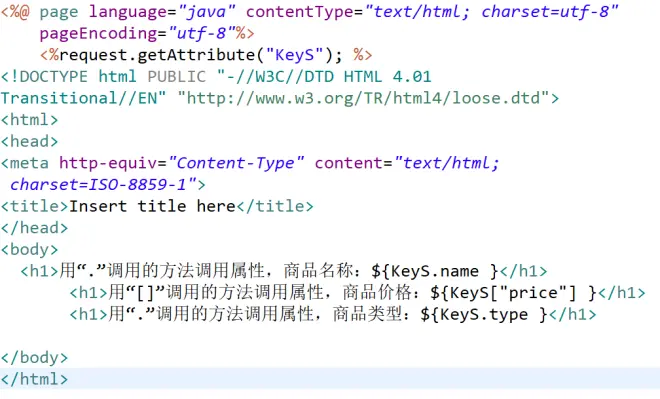
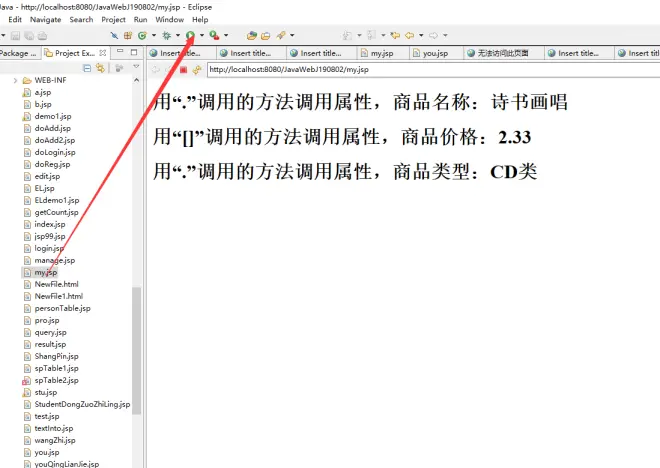
4、(难)实现页面跳转show.jsp跳转到result.jsp,根据show.jsp页面的prop值动态显示第1题中Student对象的属性值。
例如在show.jsp页面中,request.setAttribute("prop","sname");
在跳转到result.jsp页面后,显示学生的姓名值(提示:使用[]方式动态显示)
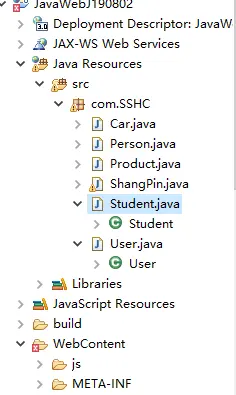

package com.SSHC;
import java.util.Date;
public class Student {
private String sname;
private String hobby;
private Date birth;
private char ssex;
private String scls;
public Student(){
}
public String getSname() {
return sname;
}
public void setSname(String sname) {
this.sname = sname;
}
public String getHobby() {
return hobby;
}
public void setHobby(String hobby) {
this.hobby = hobby;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
public char getSsex() {
return ssex;
}
public void setSsex(char ssex) {
this.ssex = ssex;
}
public String getScls() {
return scls;
}
public void setScls(String scls) {
this.scls = scls;
}
public Student(String sname, String hobby,
Date birth, char ssex, String scls) {
super();
this.sname = sname;
this.hobby = hobby;
this.birth = birth;
this.ssex = ssex;
this.scls = scls;
}
@Override
public String toString() {
return "Student [sname=" + sname +
", hobby=" + hobby + ", birth=" + birth
+ ", ssex=" + ssex + ", scls=" + scls + "]";
}
}
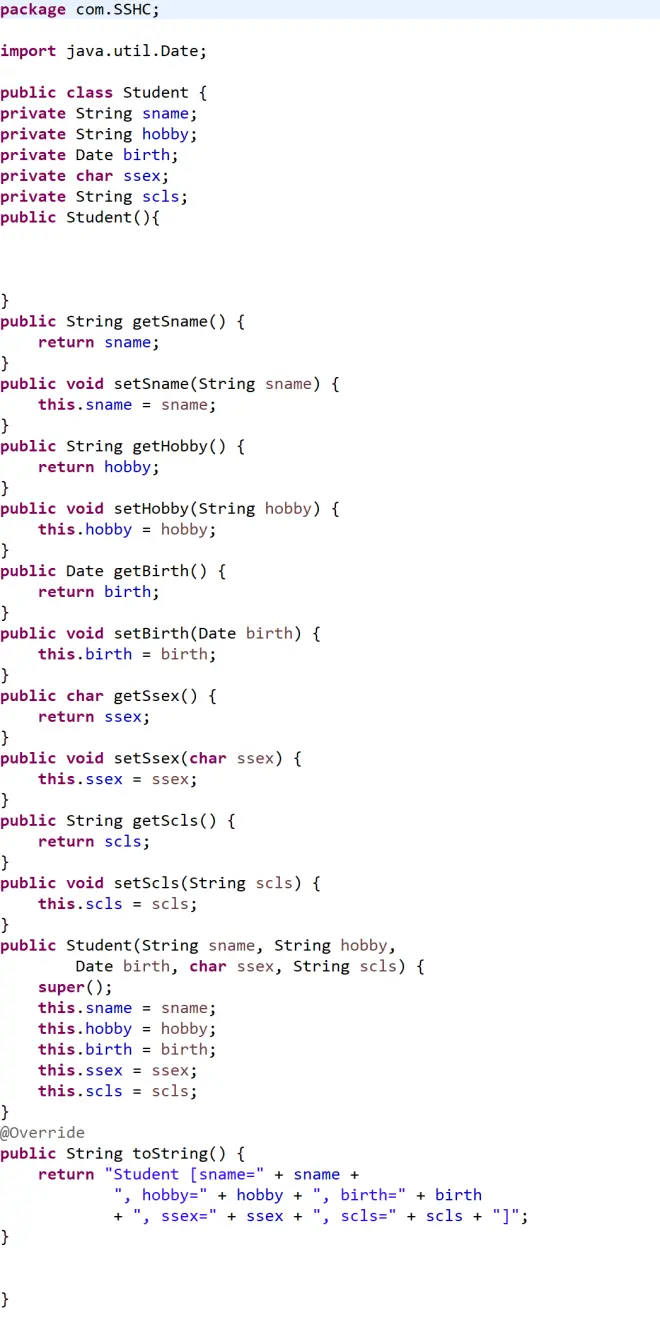
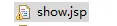
<%@page import="com.SSHC.Student"%>
<%@page import="java.util.Date"%>
<%@ page language="java" contentType="text/html;
charset=UTF-8" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"
+request.getServerName()+":"+request.getServerPort()+path+"/";
Student S = new Student();
S.setSname("诗书画唱");
S.setHobby("做视频和编程");
//下面的Date的类型要声明为“X/X/X”的格式,不然会报错等。
Date D= new Date("2000/7/20");
S.setBirth(D);
S.setSsex('男');
S.setScls("诗书画唱班");
request.setAttribute("KeyS", S);
request.getRequestDispatcher("result.jsp")
.forward(request, response);
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base hreff="<%=basePath%>">
<title></title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content=
"keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
</body>
</html>
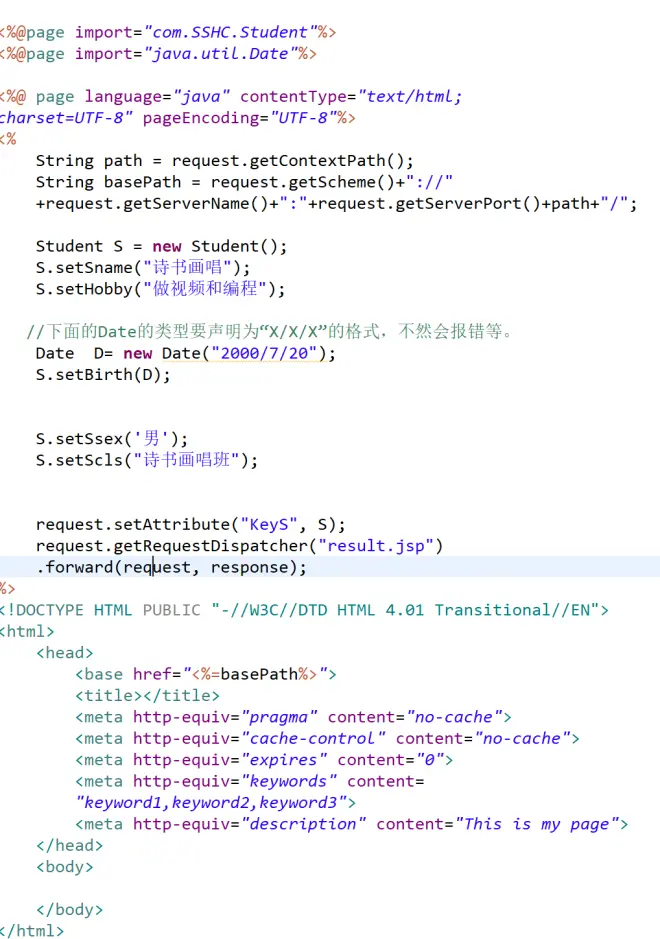

<%@page import="com.SSHC.Product"%>
<%@ page language="java" contentType="text/html;
charset=UTF-8" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"
+request.getServerName()+":"+request.getServerPort()+path+"/";
Object obj = request.getAttribute("KeyS");
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base hreff="<%=basePath%>">
<title></title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,
keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<h1>用“[]”调用的方法调用属性,姓名:${KeyS["sname"] }</h1>
</body>
</html>
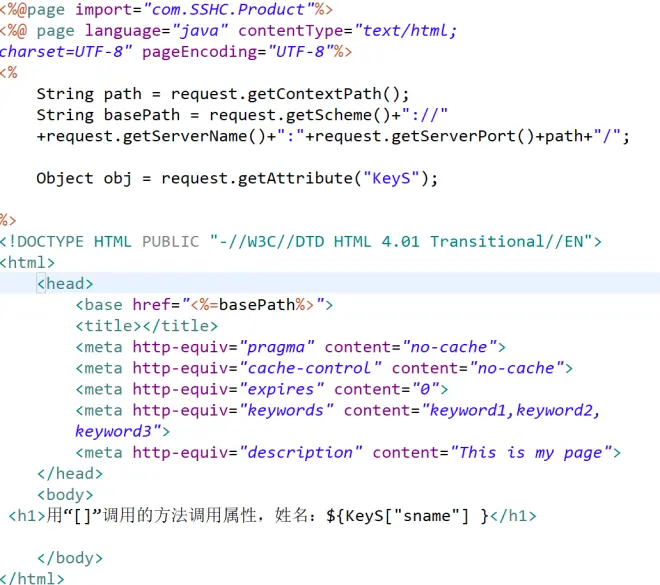
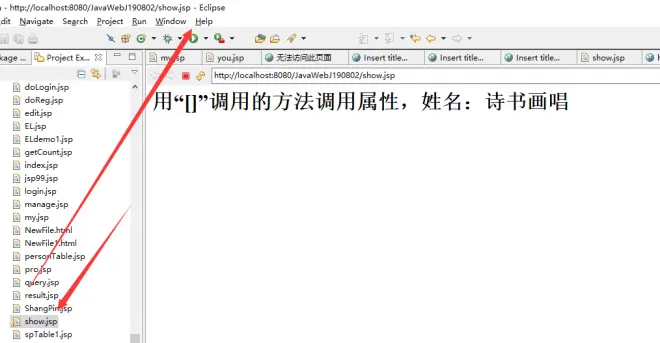
5、在第1题中,在a.jsp页面中传递一个message到b.jsp页面,在b.jsp页面中通过EL表达式和三元运算符进行显示:如果message的内容是success,则显示登录成功,否则显示登录失败。

<%@page import="com.SSHC.Student"%>
<%@page import="java.util.Date"%>
<%@ page language="java" contentType="text/html;
charset=UTF-8" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"
+request.getServerName()+":"+request.getServerPort()+path+"/";
Student S = new Student();
S.setSname("诗书画唱");
S.setHobby("做视频和编程");
//下面的Date的类型要声明为“X/X/X”的格式,不然会报错等。
Date D= new Date("2000/7/20");
S.setBirth(D);
S.setSsex('男');
S.setScls("诗书画唱班");
request.setAttribute("KeyS", S);
String message="success";
request.setAttribute("KeyM", message);
request.getRequestDispatcher("b.jsp")
.forward(request, response);
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base hreff="<%=basePath%>">
<title></title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content=
"keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
</body>
</html>
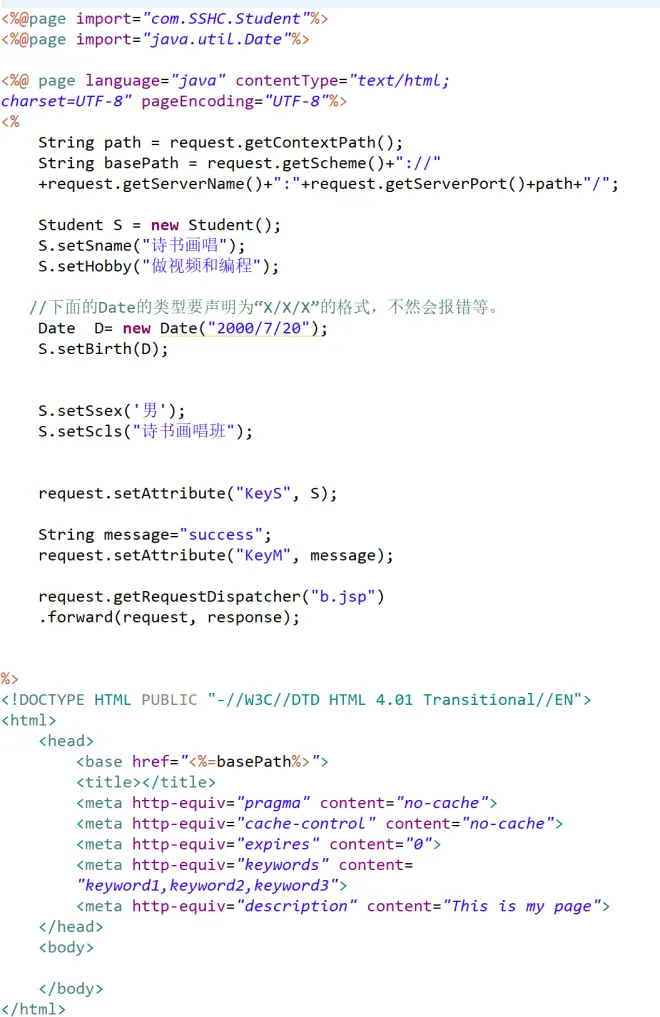
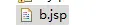
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<%request.getAttribute("KeyS");
request.getAttribute("KeyM");%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01
Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;
charset=ISO-8859-1">
<title>Insert title here</title>
</head>
<body>
方法一(用equals判断相当):<br>
${KeyM.equals("success")?"登录成功":"登录失败" }<br>
方法二(用==判断相当):<br>
${KeyM=="success"?"登录成功":"登录失败" }
<h1>用“.”调用的方法调用属性,姓名:${KeyS.sname }</h1>
<h1>用“[]”调用的方法调用属性,爱好:${KeyS["hobby"] }</h1>
<h1>用“.”调用的方法调用属性,生日:${KeyS.birth }</h1>
<h1>用“[]”调用的方法调用属性,性别:${KeyS["ssex"] }</h1>
<h1>用“.”调用的方法调用属性,班级:${KeyS.scls }</h1>
</body>
</html>
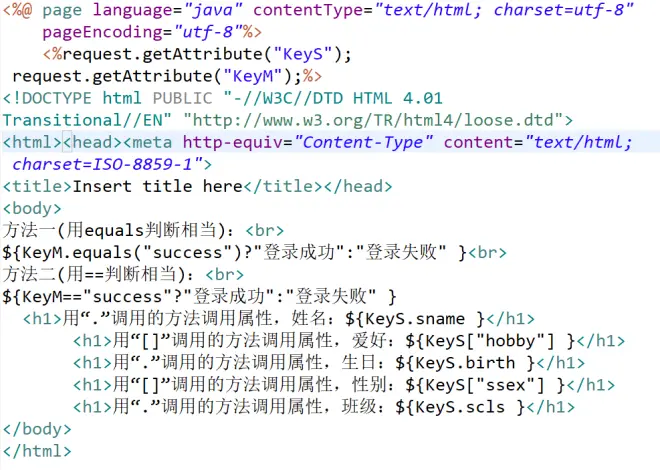
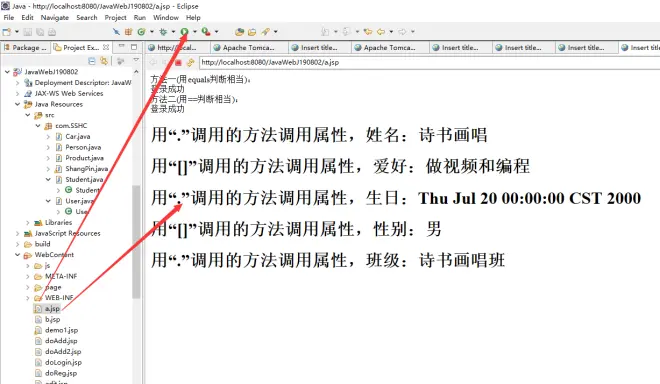
下面是要学习的内容,可以当作学习路线等提前去预习等:
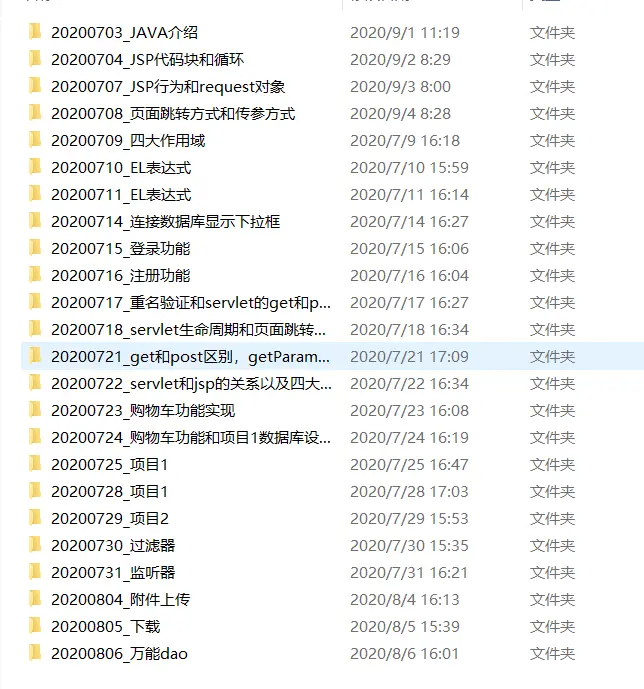

自己写的题目:
用上EL表达式,三元表达式,直接调用,“."或”[]“调用类的属性值等
下面是自己写的答案和超有用得个人的总结等:
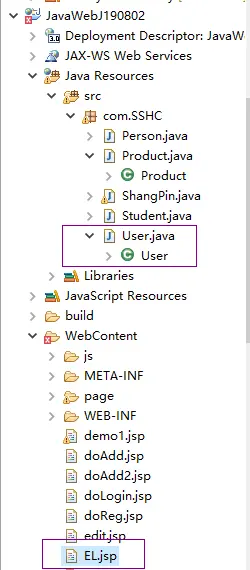
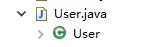
package com.SSHC;
public class User {
private String act;
private String pwd;
public String getAct() {
return act;
}
public void setAct(String act) {
this.act = act;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
}
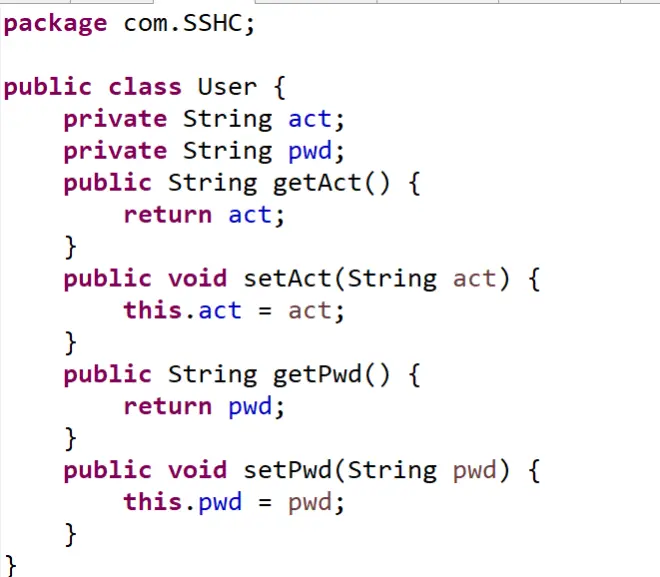

<%--
下面是用import导入包 --%>
<%@page import="com.SSHC.User"%>
<%@ page language="java" contentType="text/html;
charset=UTF-8" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"
+request.getServerName()+":"+request.getServerPort()+path+"/";
/*
下面是先声明一个num的值为66,之后给num取名字为"_num"。
msg,flag也类似,都用了setAttribute方法。
只不过
msg,flag的作用域为request,而
num的作用域为application。
application
[ˌæplɪˈkeɪʃn]
n. 申请; 请求;
*/
//————————
int num =66;
application.setAttribute("_num", num);
//——————
String msg = "关注诗书画唱并给三连!";
request.setAttribute("_msg", msg);
//——————
boolean flag = true;
request.setAttribute("_flag", flag);
//——————
/*
下面是声明了一个User类,用上
new,就是把User类调用出来。
之后用一个变量接收,
而要想接收User类的话,就可以通过把
(可统一变的)别名u的数据类型
声明为User。
之后可以通过用setXXX方法给User的各个属性等
赋值。最后将u对象放到作用域 request的
setAttribute方法中去,命名为"_user",
以便同过"_user"使用EL表达式:
*/
User u = new User();
u.setAct("诗书画唱");
u.setPwd("666");
request.setAttribute("_user", u);
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base hreff="<%=basePath%>">
<title></title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content=
"keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<%--
自己对EL表达式的总结:
先用上XXX.setAttribute("YYY",ZZZ)。
XXX就是request等的作用域。
"YYY"就是别名,类似于Key键,
ZZZ类似于Value。
总之如果ZZZ="Value",那么通过
${YYY}在HTML网页界面调用的值就是
Value。
如果直接${YYY},ZZZ没被赋值,
那么在网页打印的内容就是空白,什么都没有,而不是
Null等内容。
——————
如果"YYY"为类的别名,
那么可以通过${YYY.类的属性名}
或${YYY[类的属性名]}调用。
这些都是JS中的调用方法。
——————————
下面就是EL表达式的调用:
--%>
<h1>直接调用:${_num }</h1>
<h1>直接调用:${_msg }</h1>
<!--
运用设计:
如果flag为false就显示未登录,如果flag为true,就显示注销 。
三元表达式(也就是三元运算符,三目表达式,
总之我这些叫法我都听别人讲过。
自己总结的三元表达式的语法:
A?B:C(就是A为布尔型,boolean ,已经声明为true或flase
当 A为true时显示B,B起作用。 当 A为flase时,C起作用。
)
-->
<h1>用三元表达式调用:${_flag ? "注销" : "未登录" }</h1>
<!--
_user.act中的act就是User类中的属性名
-->
<h1>用“.”调用的方法调用属性:${_user.act }</h1>
<h1>用“[]”调用的方法调用属性:${_user["act"] }</h1>
<h1>用“.”调用的方法调用属性:${_user.pwd }</h1>
<h1>用“[]”调用的方法调用属性:${_user["pwd"] }</h1>
</body>
</html>
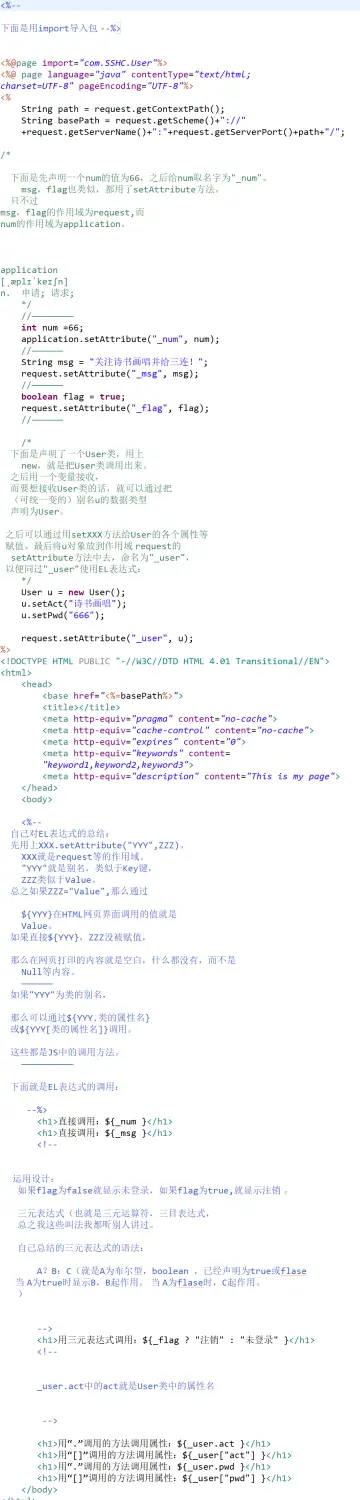

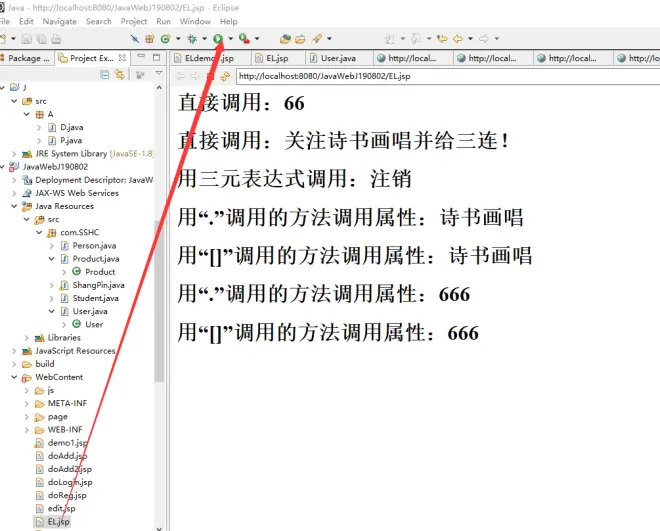
______
扩展知识:
EL表达式
EL表达式就是JSP中的一种特有的语言,可以简化我们的java代码。
EL表达式写法: ${表达式},注意: {}中间只能是表达式,不能是语句。
EL表达式有两种运算符: . 和[] (JS对象的运算符)
EL表达式可以写在JSP页面的任何地方。
EL表达式中的变量的显示过程:会依次从pageContext, request, session以及
applicaion四个作用域中找这个变量, 一旦找到了就返回这个值,如果四个作用域都
找不到,就显示为”" (不是显示为null)。
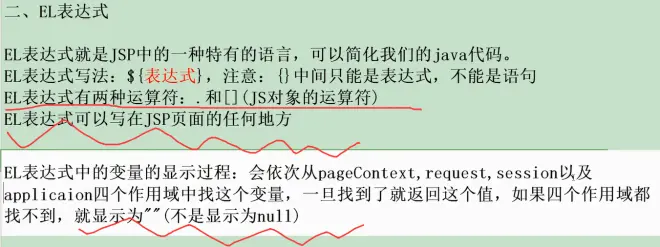
application
[ˌæplɪˈkeɪʃn]
n. 申请; 请求;
__________
JSP四大作用域分别为:
page, request ,session, application 。
第一个作用域是page,他只在当前页面有效,也就是用户请求的页面有效,当当前页面关闭或转到其他页面时,page对象将在响应回馈给客户端后释放。
第二个作用域是request,他在当前请求中有效,request可以通过setAttribute()方法实现页面中的信息传递,也可以通过forward()方法进行页面间的跳转,需要注意的是request是转发不是重定向,转发相对于浏览器来说是透明的,也就是无论页面如何跳转,地址栏上显示的依旧是最初的地址。
第三个作用域是session,他在当前回话中有效。当一个台电脑上的同一浏览器对服务器进行多次访问时,在这多次访问之间传递的信息就是session作用域的范围。它从浏览器发出第一个HTTP请求即可认为会话开始,但是会话结束的时间是不确定的,因为在浏览器关闭时并不会通知服务器,一般Tomcat设置的默认时间为120分钟,也可以通过setMaxInactiveInterval(int)方法进行设置,或是通过invalidate()方法强制结束当前会话。
第四个作用域是application,他在所有的应用程序中都有效,也就是当服务器开始到服务器结束这段时间,application作用域中存储的数据都是有效的,同样可以通过setAttribute赋值和getAttribute取值。
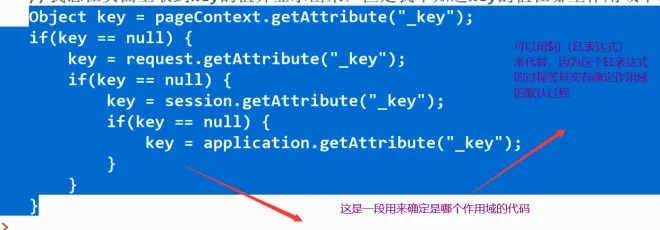
